Python Script for Verifying Internet Connectivity
Written on
Introduction
This Python script is designed to determine the internet connectivity status of a system or network. It can be particularly beneficial in several scenarios, such as:
- Automated Testing: In cases where automated tests require an internet connection, this script can verify connectivity before executing the tests. If the internet is down, it can halt the tests and report the problem.
- Monitoring: The script can periodically check for internet connectivity, sending alerts if a disconnection occurs. This feature is useful for maintaining the internet status in various networks or systems.
- Diagnostics: When an application is malfunctioning, and you suspect connectivity issues, this script can swiftly verify the internet connection status and suggest potential solutions if disconnected.
- Scripting: The script can be integrated into larger automation processes, helping determine whether to proceed based on the connectivity status.
Overall, this script is a versatile tool for assessing internet connectivity and taking appropriate actions based on its findings.
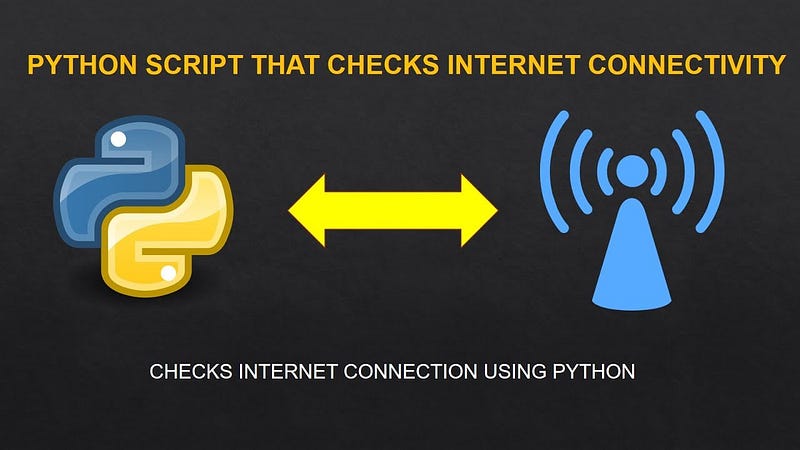
Understanding the Python Script
This script employs try-except blocks to handle various exceptions, providing error messages along with potential solutions. It incorporates the sys library to manage exit statuses.
- Exit Status Codes:
- sys.exit(0): Indicates successful execution with internet connectivity.
- sys.exit(1): Indicates execution completion with no internet connection.
You can verify the exit status using the special shell variable $?. A value of 0 signifies an active connection, while 1 indicates a disconnection.
Script Breakdown
- Import Libraries:
- import requests: Enables HTTP requests to websites.
- import socket: Catches socket-related exceptions.
- import sys: Facilitates interaction with the system.
- Function Definition:
- def check_internet_connectivity(): This function checks for internet connectivity by attempting to connect to a specific website, such as Google.com.
- requests.get('http://www.google.com', timeout=5): Makes a GET request to Google. If successful, it returns True, indicating connectivity. A timeout of 5 seconds is set to avoid prolonged waiting.
- Error Handling:
- except requests.ConnectionError as e: Catches connection errors and prints an error message.
- print(f"Loss of connectivity: {e}"): Displays the reason for the loss of connection.
- print("Possible fixes:"): Suggests potential solutions.
- return False: Returns False, indicating no connectivity.
- Further Exception Handling:
- Additional except blocks handle socket.timeout, requests.ConnectTimeout, and requests.ReadTimeout exceptions.
- Final Connectivity Check:
- After executing check_internet_connectivity(), the script checks the returned value:
- If True, it prints "Internet is connected." and exits with a status of 0.
- If False, it prints "Internet is not connected." and exits with a status of 1.
- After executing check_internet_connectivity(), the script checks the returned value:
It’s important to note that the script will always print error messages and suggested fixes, regardless of the exit status.
Chapter 1: Practical Application
The first video titled "Python Tutorial | How to check if your PC has internet connection" provides a detailed demonstration of how to implement the connectivity check in Python.
Chapter 2: Advanced Techniques
The second video, "Internet Connection Checker - Python," discusses advanced methods for checking internet connectivity using Python scripts.
Conclusion
Thank you for reading! I hope you found this information valuable. If you have any questions or need further assistance, feel free to reach out. 😊